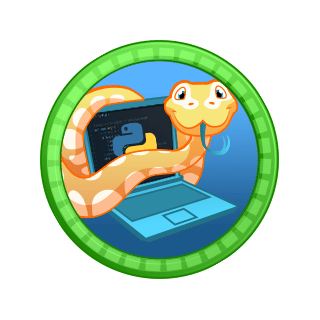
Welcome to Python
Get introduced to Python. Learn Python syntax, display output, and write correct programs.
7 Modules:
- Introduction
- Output
- Functions and Function Calls
- Arguments
- Syntax
- Comments
- Quiz
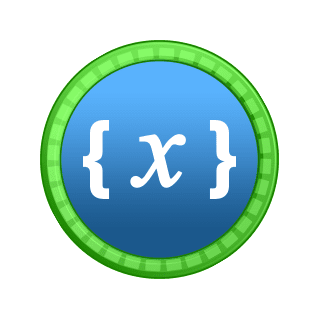
Variables and I/O
Learn to use variables to store and retrieve data in Python.
7 Modules:
- Variables
- Variable Names
- Using Variables
- Combining Values
- Input Function
- Multiple Assignments
- Quiz
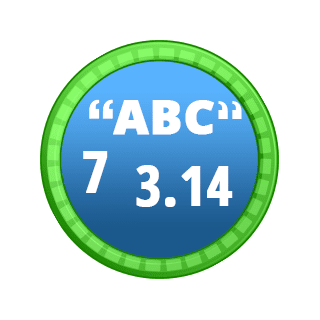
Data Types
Get introduced to Python data types such as strings, numbers, and booleans.
9 Modules:
- Data Types
- Concatenation
- Integers
- Floats
- Booleans
- Type Error
- Str Function
- Int Function
- Quiz
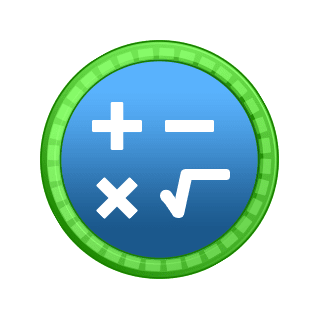
Math Operators
Learn to use math operators such as addition, multiplication, division.
11 Modules:
- Addition and Subtraction
- Multiplication and Division
- Floats
- Float Function
- Exponents
- Modulus
- Order of Operations
- Increment and Decrement
- More On Increment and Decrement
- Fibonacci Sequence
- Quiz
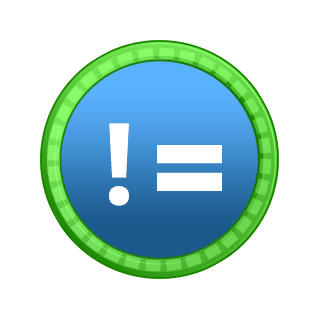
Boolean Logic
Use boolean operators to evaluate logical expressions in Python.
8 Modules:
- Logic
- Equality Operators
- Inequalities
- Comparing Strings
- And Operator
- Or Operator
- Not Operator
- Quiz
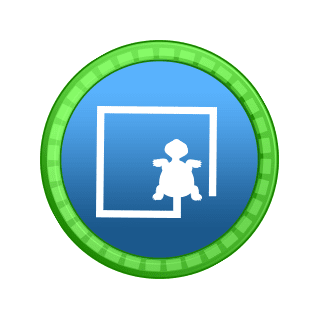
Turtle Graphics
Use the turtle library to draw shapes. Create graphics and animations.
9 Modules:
- Libraries
- Import Syntax
- Other Libraries
- Turtle Library
- Draw a Scene
- Turtle Geometry
- Filling In Shapes
- Turtle Speed
- Create a Scene
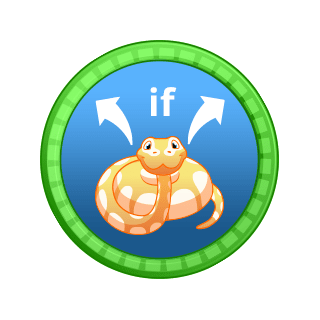
Branching
Learn to use conditions in if, elif, and else statements to control the flow.
9 Modules:
- If Statements
- If Statement Syntax
- Conditions
- Else Statements
- Else Statement Syntax
- Elif Statements
- Nested If Statements
- Play Against a Computer
- Quiz
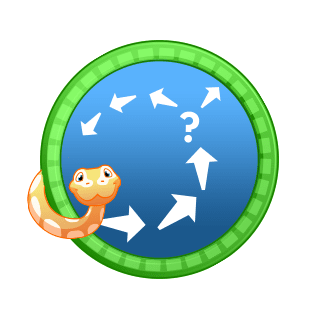
While Loops
Use conditional while loops to iterate through instructions.
8 Modules:
- While Loop
- Components of a While Loop
- Repeating Instructions
- Accumulating Results
- Branching In A While Loop
- Break
- Continue
- Quiz
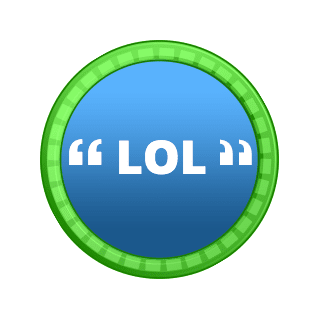
Strings
Learn to handle text and manipulate strings in Python.
9 Modules:
- Strings
- String Indexes
- Index Ranges
- String Functions
- Counting Instances
- String Operators
- Finding the Index
- String Formatting
- Quiz
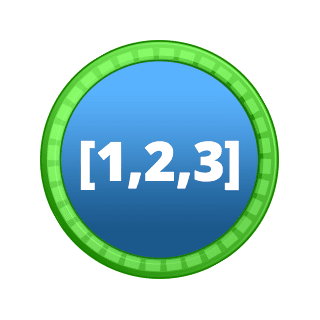
Lists
Use list data type to store a sequence of values in Python.
15 Modules:
- Lists
- Searching a List
- List Indexes
- Index Ranges
- Changing Elements
- List Iteration
- Statistics
- Comparing Adjacent Elements
- Binary Search
- List Operations
- Adding To A List
- Removing From A List
- Ordering A List
- Functions With List Arguments
- Quiz
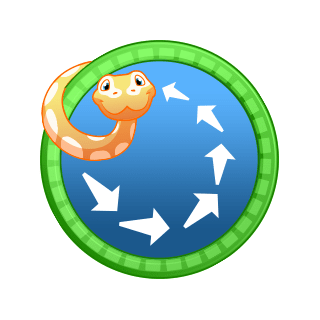
For Loops
Use for loops to repeat instructions and iterate over lists.
9 Modules:
- For Loops
- Iteration Variable
- Iterating On Strings
- Number Of Iterations
- Range Function
- Iterating On Indexes
- Calendar Loops
- Printing Shapes
- Quiz
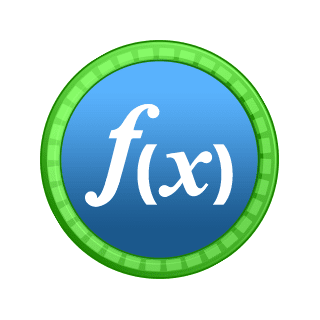
Functions
Define custom functions to create more structured programs.
8 Modules:
- Functions
- Multiple Functions
- Parameters
- Return Values
- Default Parameters
- Global Variables
- Testing Functions
- Quiz
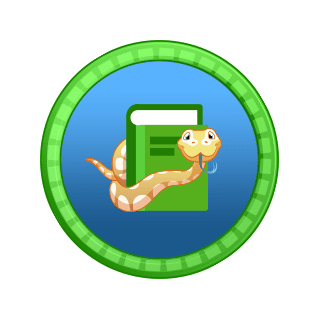
Dictionaries
Use Python dictionaries to store a collection of values accessible by name.
6 Modules:
- Dictionaries
- Accessing a Value
- Adding Key-Value Pairs
- Dictionary Iteration
- Keys and Values
- Quiz
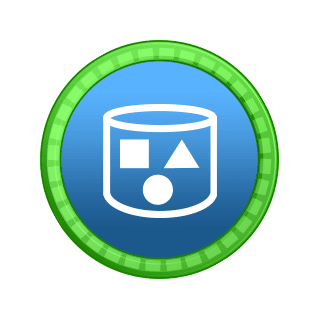
Classes and Objects
Learn to define and organize collections of functions and data as objects and classes.
7 Modules:
- Classes And Objects
- Define A Class
- Attributes
- Constructor
- Parameters
- Multiple Classes
- Quiz
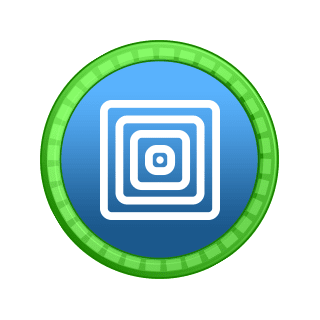
Recursion
Learn about recursion and use it as a problem-solving technique.
6 Modules:
- Recursion
- Multiple Base Cases
- Recursion and Lists
- Fractals
- Helper Function
- Quiz