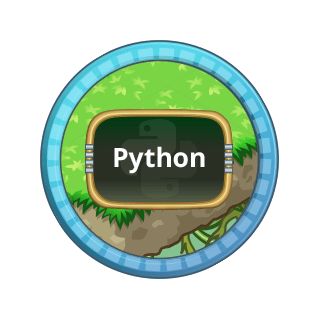
The Basics
Get acquainted with the basics of Python programming.
14 Modules:
- Welcome
- Course Overview
- Jungle Run
- More Dust
- Around the Corner
- Scale the Slopes
- Stairway to Safety
- What are Comments?
- Around the Bend
- Bunny Hop
- Naming Conventions
- Left-Right
- Review
- Quiz
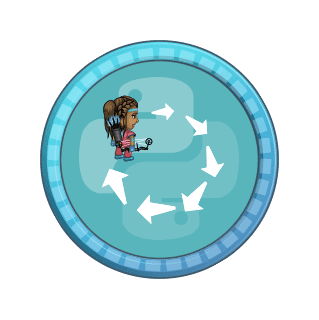
Loops and Patterns
Learn how to represent repeating patterns with code.
14 Modules:
- Path to Safety
- What are For Loops?
- What is Indentation?
- What are Arithmetic Operators?
- Forward, Fire, Repeat
- Make a Zig-Zag
- March On
- Jump, Forward
- Forward, Jump
- Up and Down
- What are Nested Loops?
- U-Turn
- Review
- Quiz
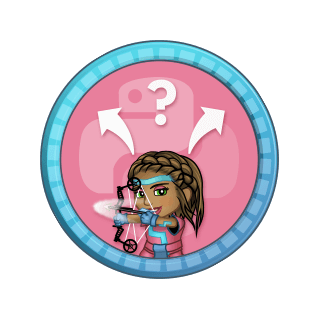
Conditional Logic
Explore boolean values and boolean logic. Write programs that "make decisions."
16 Modules:
- What Are Conditionals?
- Path Right
- Path Left
- More Turns!
- What Are Assignment Operators?
- What Are Comparison Operators?
- What Are Logical Operators?
- Hazardous Path
- What Is an Else?
- Death Valley
- Traps
- What Are Nested Ifs?
- G for Grandeur
- Silly Path
- Review
- Quiz
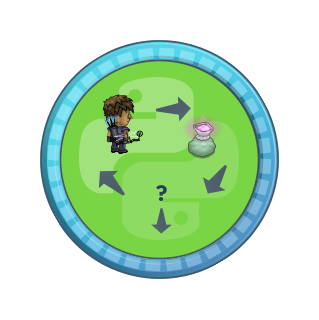
Conditional Loops
Write loops that repeat instructions, depending on whether a condition holds true.
12 Modules:
- What Is a While Loop?
- Detect a Path
- Forward, Left
- Right, Forward
- What is Break?
- While Else
- Path Ahead?
- Left Spiral
- Right Spiral
- Choose a Path
- Review
- Quiz
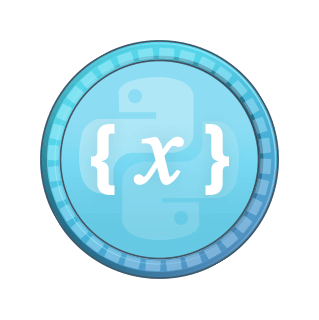
Variables
Learn about variable initialization, declaration, and more.
15 Modules:
- What Are Variables?
- Fuel Up
- Stockpile
- Rocket Jump
- What Are Strings?
- Hack the Trap 1
- How Do You Define Multiple Variables?
- Let Loose
- It's a Toughie
- Hack the Trap 2
- Welcome to the Jungle
- Obstacle Course
- Hack the Trap 3
- Review
- Quiz
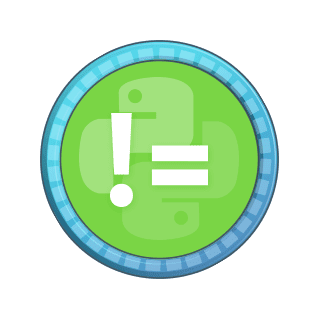
Expressions
Learn how operators can be used to form expressions.
11 Modules:
- What Are Expressions?
- Trickier Trap 1
- Trickier Trap 2
- What Are Numbers?
- What is Operator Precedence?
- Trickier Trap 3
- Trickier Trap 4
- What Are Lists?
- What Are Dictionaries?
- Review
- Quiz
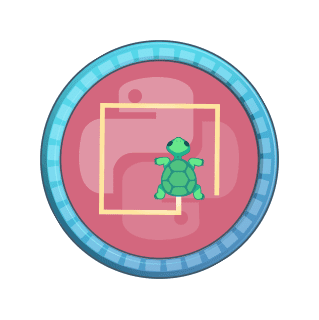
Using the Turtle Tool
Create graphics with the Turtle module.
7 Modules:
- The Turtle API
- Setting up the Screen
- Creating Turtles
- Moving and Drawing with the Turtle
- Turtle Graphing
- Review
- Quiz
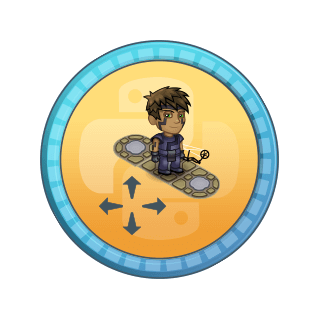
User Interaction
Learn to set up and handle keyboard and mouse events in Python.
6 Modules:
- Writing Your Own Functions
- Key Detection
- Adding a Delay with Timers
- Asteroids
- Review
- Quiz
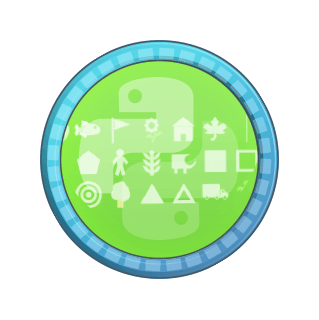
Game Design
Learn about game loops, win/loss conditions, and keeping score in Python.
6 Modules:
- What Is a Sprite?
- What Are Game Loops?
- More on Lists
- More on Dictionaries
- Review
- Quiz
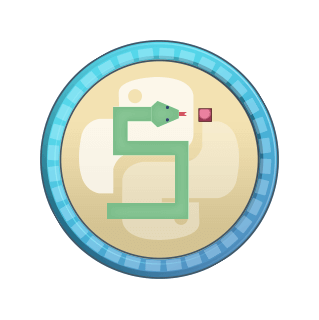
Snake
Create a "snake" game on your own.
4 Modules:
- Make A Game: Snake
- Snake: Setting Up the Game
- Create the Game
- Completing the Snake Game
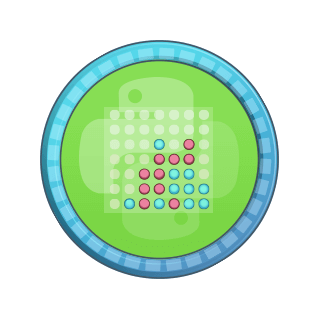
Connect 4
Build a game of "Connect 4" on your own.
4 Modules:
- Make a Game: Connect 4
- Connect 4: Setup the Board
- Connect 4: Player Controls
- Completing the Connect 4 Game
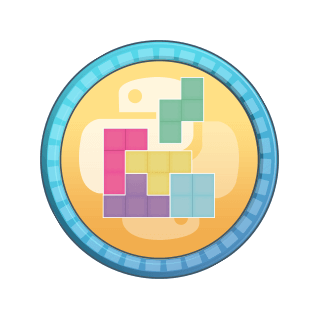
Tetris
Program a game of "Tetris" on your own.
5 Modules:
- Tetris
- Tetris: Game Setup
- Tetris: Setup Other Variables
- Tetris: Create the Display
- Tetris: Completing the Game
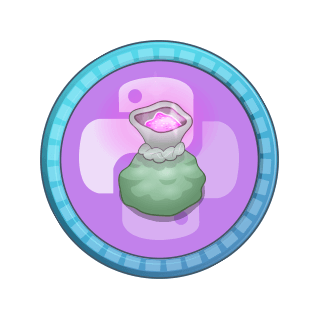
Final Game
Build a game of your choice. Choose from "Frogga" and "Pong."
2 Modules:
- Frogga
- Pong