
Unit 1: Java Basics
Get familiar with the course and explore basic Java syntax.
7 Lessons:
1. Warm Up!
2. Introduction
3. Visualizing Algorithms
4. Printing to the Console
5. Strings and Input
6. Algorithms Review
7. Algorithms Quiz

Unit 2: Abstraction and Methods
Explore methods, a way to keep code organized and repeatable.
6 Lessons:
8. Abstraction
9. More on Methods
10. Composition
11. ASCII Art Methods
12. Methods Review
13. Methods Quiz

Unit 3: Variables and Data Types
Dive deeper into the idea of variables, exploring how Java encodes information as well as the binary number system. Students will learn key concepts like variable initialization, declaration, data types, bits, bytes, and more.
7 Lessons:
14. Encoding Information
15. Binary Numbers
16. Variables
18. Types
19. Variables Review
20. Variables Quiz

Unit 4: Expressions and Operators
Perform many calculations at once using expressions and operators. In this lesson, students learn how to create their own useful expressions.
5 Lessons:
21. What’s an Expression?
22. Variable Initialization in Java
23. Working with Numbers
24. Expressions, Operators, and Statements Review
25. Expressions, Operators, and Statements Quiz

Unit 5: Objects
Learn about object-oriented programming, including the relationship between a class and an object, while creating their own custom types. In this way, students learn how object-oriented programming can make for more general solutions to problems.
9 Lessons:
26. Variables and Memory
27. Properties and Behaviors
28. Classes
29. Instance Variables and Methods
30. Constructors
31. Drawing Shapes
32. The Math Class
33. Review
34. Quiz

Unit 6: Strings
A string is any text-based information longer than a single character. For example, “Greetings” and “Game Over” are examples of strings. Explore topics like concatenation, string traversals, and more.
6 Lessons:
35. Introduction to Strings
36. String Methods
37. More String Methods
38. Substrings
39. Review
40. Quiz
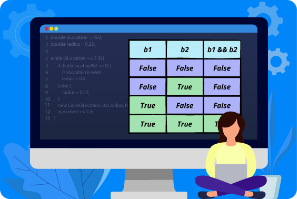
Unit 7: Booleans and If Statements
Learn about true-and-false values, digital logic, and how to make programs “make decisions” using control structures like if statements. This unit also explores the idea of selection to handle a wider variety of inputs and conditions.
7 Lessons:
41. Boolean Expression Basics
42. Logical Operators in Java
43. Using Relational and Logical Operators Together
44. Conditional Statements
45. Else and Else-If Statements
46. Conditionals Review
47. Conditionals Quiz

Unit 8: While Loops
In a while loop, code is repeated, as long as some condition is true. This approach allows for flexibility in responding to a changing situation. Explore how to take control of loops while solving drawing puzzles.
8 Lessons:
48. The While Loop
49. Take Control of Loops
50. Infinite Loops
51. Draw with Loops
52. Randomness
53. While Loop Puzzles
54. Review
55. Quiz

Unit 9: For Loops
Explore another common way to iterate through code: the for loop. This construct is a convenient way to repeat when you know how many times you want a block of code to be executed. Students also explore accumulating, filtering, and mapping, using some classic CS brainteasers like FizzBuzz.
7 Lessons:
56. For Loop Basics
57. Nested Loops
58. Accumulating, Filtering, Mapping
59. Loop Challenges
60. Common Pitfalls
61. Review
62. Quiz

Unit 10: Algorithms Challenge Lab
Get familiar with so-called “Standard Algorithms” with some more hands-on practice. Experiment with efficiency and informally compare algorithm speed.
1 Lesson:
63. Lab: Algorithms

Unit 11: Classes
A deeper dive into object-oriented programming, covering topics like classes, data, constructors, and methods.
12 Lessons:
64. Constructors
65. “this”
66. Intangible Objects
67. Adding Functionality
68. toString
69. Separation of Concerns
70. Setters and Getters
71. Writing Setters and Getters
72. National Parks
73. Impact of Computing
74. Review
75. Quiz

Unit 12: Array Basics
Learn about the array, which can be used to manage large amounts of data in a single variable. Also learn about array operations and how torprocess the data found in arrays using selection and iteration.
7 Lessons:
76. The Case for Arrays
77. Creating Arrays
78. For Loops and Arrays
79. Processing Arrays
80. Taking Flight
81. Arrays Review
82. Arrays Quiz

Unit 13: Array Applications
Explore practical applications of arrays, as well as additional methods for array processing. For example, use arrays to explore global temperature data from NASA and create simple bar charts to visualize patterns in the data.
11 Lessons:
83. Arrays and Objects
84. Comma Separated Values
85. Arrays and Objects 2
86. ToDo List
87. Dream Journal
88. Global Temperatures
89. Graphing Temperatures
90. Regional Temperatures
91. ForEach Loops
92. Arrays and Objects Review
93. Arrays and Objects Quiz
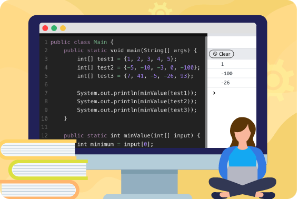
Unit 14: Array Challenge Lab
Students practice with a sequence of Array programming challenges, which will require them to invent their own algorithms for array traversal and processing.
1 Lesson:
94. Lab: Array Challenges

Unit 15: Inheritance
Explore the idea of class hierarchy and inheritance patterns with hands-on exercises using superclass and subclass.
7 Lessons:
95. Introduction to Inheritance
96. Extends
97. Equals
98. Super
99. Polymorphism
100. Inheritance Review
101. Inheritance Quiz

Unit 16: Capstone Project
In this scaffolded but open-ended prompt, students will develop their own original program, making choices about the purpose of their program and what its functionality will be. After several work periods, students will take a break and write about the program they made, as well as the lessons they learned.
4 Lessons:
102. Capstone Project, Day One
103. Day Two: Find Bugs
104. Day Three: Minimum Viable Product
105. Day Four: Polish, Test, and Document